Request throttling in .NET Core MVC
Security in apis are important and we might not want the apis we build to be overly used. I built a small attribute function that allows for throttling of a specific endpoint.
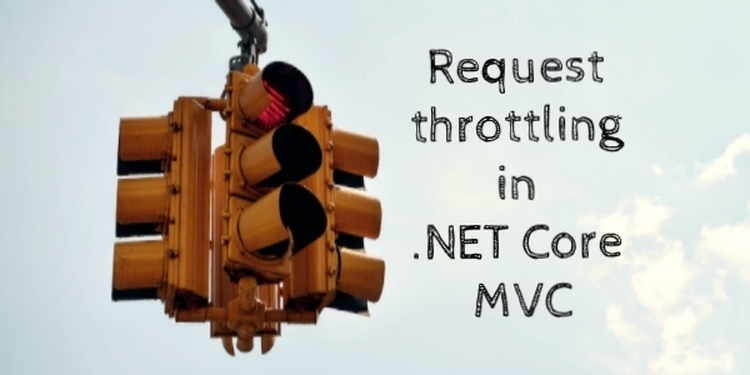
Why do I need to throttle usage?
Security in apis are important and we might not want the apis we build to be overly used. This could be to prevent DDoS attacks or to make sure no one tries to brute-force-use your api. To solve this problem I built a small attribute function that allows for throttling of a specific endpoint.
When I started writing this i got alot of inspiration from this post on stack overflow.
Attribute code
Here is the code for the throttling attribute
[AttributeUsage(AttributeTargets.Method)]
public class ThrottleAttribute : ActionFilterAttribute
{
public string Name { get; set; }
public int Seconds { get; set; }
public string Message { get; set; }
private static MemoryCache Cache { get; } = new MemoryCache(new MemoryCacheOptions());
public override void OnActionExecuting(ActionExecutingContext c)
{
var key = string.Concat(Name, "-", c.HttpContext.Request.HttpContext.Connection.RemoteIpAddress);
if (!Cache.TryGetValue(key, out bool entry))
{
var cacheEntryOptions = new MemoryCacheEntryOptions()
.SetAbsoluteExpiration(TimeSpan.FromSeconds(Seconds));
Cache.Set(key, true, cacheEntryOptions);
}
else
{
if (string.IsNullOrEmpty(Message))
Message = "You may only perform this action every {n} seconds.";
c.Result = new ContentResult {Content = Message.Replace("{n}", Seconds.ToString())};
c.HttpContext.Response.StatusCode = (int) HttpStatusCode.Conflict;
}
}
}
Example usage
[Route("api/[controller]")]
public class ActionsController : Controller
{
// GET /api/actions/throttle
[HttpGet("throttle")]
// Only allow access every 5 seconds
[Throttle(Name = "ThrottleTest", Seconds = 5)]
public object GetThrottle() => new
{
Message = "OK!"
};
}
Share this post
Twitter
Facebook
Reddit
LinkedIn